Blazor & Clean Architecture Masterclass
Learn .NET, Blazor, Clean Architecture, and advanced features with a real-world project. Build apps step-by-step with hands-on lessons that make learning fun and easy!
β About the Course
If youβre ready to master .NET, Blazor and Clean Architecture, this course is for you! The Blazor & Clean Architecture Masterclass is a step-by-step guide to building powerful, real-world applications.
Youβll start with the basics, like setting up tools and creating your first Blazor project. Then, youβll dive into advanced concepts, including Clean Architecture, Entity Framework, CQRS, and secure authentication with ASP.NET Core Identity.
By the end of this course, youβll have the skills to design, build, and deploy professional web applications. Whether youβre a beginner or want to take your skills to the next level, this course has everything you need to succeed.
π What You'll Learn
In this course, youβll learn everything you need to build amazing apps with Blazor and Clean Architecture:
- π» Set up your tools and create your first Blazor project step by step.
- ποΈ Understand how to organize your code using Clean Architecture so itβs easy to grow and maintain.
- π Work with databases using Entity Framework Core and add features like creating, updating, and deleting data.
- π Learn how to make your app faster and simpler with CQRS and the Mediator pattern.
- π Add secure logins and user roles with ASP.NET Core Identity.
- π οΈ Use Git and GitHub to save your code and share it like a pro.
- π‘οΈ Add Data Transfer Objects (DTOs) to make your app cleaner and easier to work with.
- π¨ Design a modern and responsive app using Tailwind CSS.
- π Deploy your app to Azure and make it live with automated workflows.
- π Explore advanced Blazor features like interactive rendering and faster page updates.
- π§ Refactor your app to make it faster and easier for users to enjoy.
π οΈ What You'll Build
Weβll create a blog app together! Youβll learn to build features like adding, editing, and deleting articles. Youβll also add secure logins and user roles to keep everything safe. By the end of this course, your app will look great with Tailwind CSS, be deployed to Azure, and use smart coding techniques like CQRS and Dependency Injection. This will be a professional-grade app you can be proud of!
π Course Content
Store your code with Git & GitHub β
Introduction to Clean Architecture β
The Infrastructure Layer: Database & Entity Framework β
Introduction to CQRS & the Mediator Pattern β
CRUD with CQRS & Blazor SSR (Static Server-Side Rendering) β
UX Improvements & Refactoring β
Authentication with ASP.NET Core Identity β
Authorization using Roles with ASP.NET Core Identity β
User Management: Interactivity with Blazor Server β
Article Management: Interactivity with Blazor WebAssembly β
The Interactive Auto Render Mode β
.NET 9 Update β
Minimal API β
Replace MediatR β
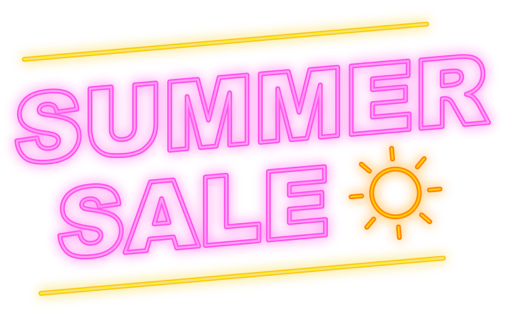
Offer ends June 26. Don't miss it!
Own This Course
$599 $299
Billed once.
- This Course Only
- 14 Hours On-Demand Videos
- Free Updates (e.g. .NET 9)
- Quizzes
- Exercises
- Source Code Download
- English Captions
- Certificate of Completion
- Lifetime Access
- Learn at Your Own Pace
Access All Courses Plan
$99$59 /month
Billed monthly.
- All Courses ($1,514 value)
- All Source Code Downloads
- Access to All Future Courses FREE
- All YouTube Tutorial Source Codes
- English Subtitles
- Certificates of Completion
- Exclusive Community Access
- Private Discord Server
- Virtual Coworking Space
- Cancel Anytime
30-Day Money-Back Guarantee
Iβm sure youβll find this course incredibly valuable. But if it doesnβt feel like the right fit, no problem! Youβre covered by a 30-day money-back guarantee β no questions asked.
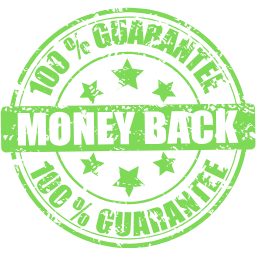
Any Questions?
Do you have some questions about the .NET Web Academy?
Feel free to send an email to mail@patrickgod.com. I'll do my best to help. π